In this next series of posts, I’m going to discuss the bitmap borders and shadows (BBS) feature that first appeared in Vista Advanced 3.5.0. If you haven’t played with them yet, you owe it to yourself to do so.
This first blog post will discuss how (and when) you can use the feature, how it works under the covers, and how to get the best possible performance from it. If you have no idea what the bitmap borders and shadows feature is, take a look at the action shot below.
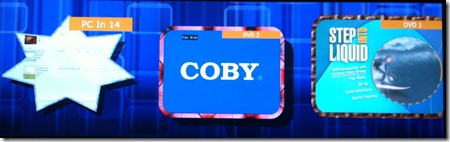
The image above shows two related features, bitmap borders and shadows and window titling. I’m going to skip over titling for this post, and concentrate only on the bitmap border / shadow feature.
What Can I do With Bitmap Borders?
Bitmap borders goes beyond the traditional supported borders to enable custom shapes and fills to be defined. Shadows applied will keep the same shape as the bitmap border, and can even have a color other than black defined (not shown).
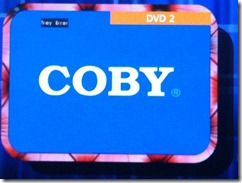 |
Features Shown:
-
Non-Rectangular window (rounded edges in this example)
-
Image file used as border texture
-
Shadow shape that matches the border shape
|
The Spyder software comes with about a dozen border shapes that you can use, as well as a number of images that can be used to texture the border. Of course you can create and use custom shapes and fill images, which I’ll be discussing in detail in the next blog post.
The KeyFrame property panel contains handles for all of the available bitmap border and shadow adjustments, and I’ve included a few screenshots of the most interesting ones below. Using them is pretty self explanatory, and so I won’t bother going through them exhaustively.
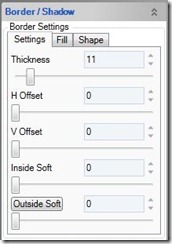 |
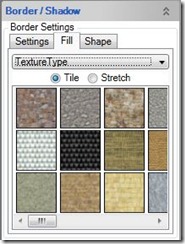 |
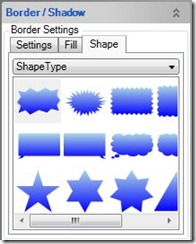 |
General Settings |
Border Texture |
Border Shape |
So How Does It Work?
Under the covers, the border / shadow settings selected are used to generate a specially formatted image at a size relative to the input video resolution, which gets loaded once into the target layer where it is in turn merged with the video each frame. The Images below show this process.
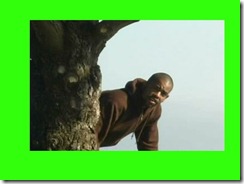 |
Video Frame Buffer (2048×1200)In this frame buffer, the active video is repositioned so that it will fit correctly when merged with the border image loaded. These offsets are programmed by the server as part of the image loading process |
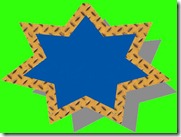 |
Bitmap Buffer (2048×1200)This buffer stores the generated image which will be merged with the video. Special bit values are encoded into the image to specify what area of the image should be mixed with the video and which area should be mixed with the VI. |
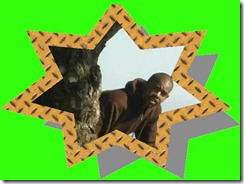 |
Resulting Image
Once the image is loaded, the hardware in the X20 layer will perform the video cutout and merge the image with the border on a frame-by-frame basis. |
Note that the image sequence above makes a point to call out the frame buffer sizes in the layer hardware; a size of 2048×1200. This is important because the generated image file previously mentioned needs to fit within these dimensions. Since the image file must be loaded relative to the input video resolution, there may not be enough ‘free’ frame buffer space to generate the desired effect.
In cases where there isn’t enough space to fit the requested bitmap border / shadow settings, the server will automatically scale back the border offset and then the border thickness until the image fits.
Also note that the image files generated can be relatively large, and in almost all cases you really need to be running a still server to ensure a good experience. While technically the bitmap borders will work when using traditional USB loading, the image load times detract much of the experience. There is additional information on the still server on the Vista forum site, and I’ve additionally just completed a post describing the still server here.
Where Bitmap Borders Won’t Work
There are a few scenarios where bitmap border functionality isn’t available, and this section attempts to list them all to save you from surprises in the field.
- – Input Resolutions above 2048 wide OR 1200 high. This causes the layers to enter a special mode that uses two layer frame buffers, and there is no bitmap border support when in this mode.
- – Running a VI height above 1850. When your running a VI at a height above 1850, certain layers cannot support still images, effectively disabling bitmap borders.
Tips for Getting the Best Experience
While certainly not required, the recommendations presented here are sure to be extremely beneficial for anyone using the bitmap border / shadow feature set.
Use a Still Server
I know I mentioned this already once in this post, but it can’t possibly be stressed enough. If your using bitmap borders and shadows (or stills for that matter), then plunk down a few hundred bucks and buy a PC to hook up to your X20 for use as a still server. The speed performance improvement when using this accessory can easily be over 10x.
Build Treatments to Recall Bitmap Border Settings
Using treatments is good practice in general, but the load times involved with adjusting bitmap borders and shadows make it an even more beneficial practice. Instead of manually adjusting KeyFrame parameters each time you want to setup a bitmap border, just make a treatment for the looks you use most frequently (border / shadow treatment settings include bitmap border options). Not only does this clean up the workflow for the operator, the on-screen experience is nicer since the system will use the smooth go processor to automatically pull the layer off screen while loading the border.
Summary
I always like knowing how things work under the hood, and if your like me then hopefully this has shed a little light on how the bitmap border and shadow feature works. In the next post, I’m going to discuss how you can create your own custom border shapes and use them with the Spyder X20.